.NET 8 has introduced new empty collections that you can use to reduce your memory usage and speed up your runtime. Often, if you want to return an empty collection such as a List<T> or Dictionary<K,T> you need to new up a new instance. The downside is memory is allocated. This might not be a big deal but then again, if that code is executed thousands of times, the memory usage and processing time adds up. Just how much? Well, it is a non-trivial amount for applications looking to maximize performance.
I created a simple .NET Core console app using the .NET 8 preview along with BenchmarkDotNet to measure the difference between the old approach vs. new.
Some example code:
[Benchmark]
public IReadOnlyDictionary<int, User> Dictionary_New()
{
return ReadOnlyDictionary<int, User>.Empty;
}
[Benchmark]
public IReadOnlyDictionary<int, User> Dictionary_Old()
{
return new Dictionary<int, User>();
}
Here are the results:
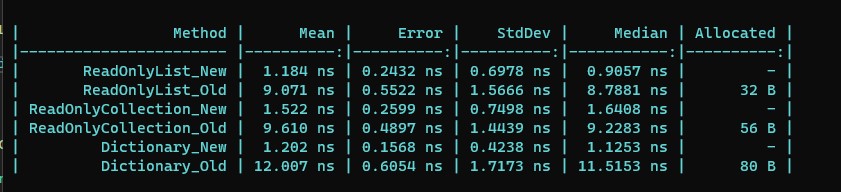
As you can see, the run time and memory usage between old and new is non-trivial and going forward, I plan to use these new empty collections.
My sample app with the full source code is available on GitHub - https://github.com/cliffordru/BenchmarkEmptyCollections
Need a developer, architect or manager? I am available - email me at [email protected]